Repetitive, time-consuming, manual tasks in Microsoft 365 take focus away from value-added work. We rounded up five PowerShell scripts that can do the trick, plus an automated solution that’s easier than writing code or doing quick fixes.
Repetitive, time-consuming tasks can frustrate anyone. They’re also a surefire way to kill creativity and cause quality decision-making to deteriorate. When managing your Microsoft 365 environment, there’s no shortage of such tasks. The solution? Automate!
For IT teams stuck in reactive mode, trying to keep up and manage the Microsoft 365 environment is no easy feat. Especially when usage grows, manual labor can wear the team out and leave zero room for proactive decision-making. Enter PowerShell and it’s handy PowerShell cmdlets.
You can automate and get a lot of unnecessary manual labor out of the way using PowerShell commands scripting language. Not only does PowerShell scripting reduce unnecessary manual labor, but it also leaves room to focus on long-term strategy. The outcome is better decision-making and effective management of your Microsoft 365 environment.
Here, we’ll look at 5 examples on how to write PowerShell scripts that kickstart your automation process. Implementing these scripts will bring your IT team closer to proactive rather than reactive mode. Here we go!
Table of contents
Learn these PowerShell scripts to enhance your administrative tasks in Microsoft 365
1. How to assign a site collection administrator in SharePoint Online using PowerShell
With the traditional route, system administrators can manually add a site collection admin in SharePoint, such as through the Microsoft 365 admin center or the SharePoint admin center. But what if you want users to automatically become site collection administrators when they connect to a site? With traditional methods, there’s no way. To automate the process, PowerShell comes to the rescue!
Steps for assigning a site collection administrator in SharePoint Online
Let’s look at an example script to see how this can be done:
Step 1: Start by assigning variables in SharePoint as follows:
$AdminURL = "sharegate-admin.sharepoint.com/"
$AdminName = "[email protected]"
$SiteCollectionURL = "https://ShareGate.sharepoint.com/sites/marketing/"
$SiteCollectionAdmin = "[email protected]"
Step 2: Next, define a username and password to connect to the site.
$SecurePWD = ConvertTo-SecureString "newpassword" -asplaintext -force
$Credential = new-object -typename System.Management.Automation.PSCredential -argumentlist $AdminName, $SecurePWD
Step 3: Now, connect to SharePoint Online.
Connect-SPOService -url $AdminURL -credential $Credential
Step 4: Finally, add the site collection admin, and you’re done!
Set-SPOUser -site $SiteCollectionURL -LoginName $SiteCollectionAdmin -IsSiteCollectionAdmin $True
Skip the PowerShell script and take your SharePoint automation further
ShareGate provides out-of-the-box automation for managing Microsoft 365. Use it to add site collection administrator rights on-site from a tenant or central administration through the PowerShell command prompt. You can even provision and populate OneDrive for business. Check out our PowerShell documentation about adding site collection administrators in SharePoint using ShareGate.
2. Export a list of all SharePoint site collections
With the traditional route, you can export a SharePoint site using Central Administration. But you can only do one at a time. Of course, if you want to get all SharePoint site collections, this process is time-consuming and redundant. You can automate the entire process by running a Windows PowerShell script.
Steps for exporting the list of all SharePoint site collections
Here’s a step-by-step PowerShell tutorial that explains how to do this:
Step 1: Start by loading assemblies in SharePoint Online.
Add-Type -Path "C:\Program Files\Common Files\Microsoft Shared\Web Server Extensions\16\ISAPI\Microsoft.SharePoint.Client.dll"
Add-Type -Path "C:\Program Files\Common Files\Microsoft Shared\Web Server Extensions\16\ISAPI\Microsoft.SharePoint.Client.Runtime.dll"
Step 2: Next, define all relevant variables.
$AdminUrl = "https://sharegate-admin.sharepoint.com/"
$UserName= "[email protected]"
$Password = "This is my password"
Step 3: Next, set up credentials to connect to the site.
$SecurePassword = $Password | ConvertTo-SecureString -AsPlainText -Force
$Credentials = New-Object -TypeName System.Management.Automation.PSCredential -argumentlist $UserName, $SecurePassword
Step 4: Now, connect to the service.
Connect-SPOService -Url $AdminUrl -Credential $Credentials
Step 5: Using the Get-SPOSite command, you can export all site collections in PowerShell.
$SiteColl = Get-SPOSite
Step 6: Finally, run a ForEach loop to get all site collections.
ForEach($Site in $SiteColl)
{
Write-host $Site.Url
}
Use ShareGate to export important SharePoint reports
Check out our PowerShell script documentation about exporting in SharePoint using ShareGate to find out what else you can pull off.
3. Use PowerShell to copy a team
You can create a copy of a team using PowerShell using the ‘Copy-MgTeam’ command. This also creates a correlated group with the admin specifying which part of a team should be copied. Let’s look at a PowerShell scripting tutorial to understand this better.
Steps to use PowerShell to copy a team
Step 1: Start by importing the Microsoft.Graph.Teams module
Import-Module Microsoft.Graph.Teams
Step 2: Next, set the parameters.
$params = @{
DisplayName = "Library Assist"
Description = "Self help community for library"
MailNickname = "libassist"
PartsToClone = "apps,tabs,settings,channels,members"
Visibility = "public"
}
Step 3: Finally, use the Copy-MgTeam cmdlet to make the copy.
Copy-MgTeam -TeamId $teamId -BodyParameter $params
More options for using PowerShell for copying teams, channels, and whatever else
With PowerShell, there are tons of copying options available. You can run scripts to copy teams, teams channels, chats, and much more! Check out our PowerShell syntax documentation about copying using ShareGate for more information.
4. Copy chats from one Microsoft 365 tenant to another using PowerShell
There’s no out-of-the-box solution to copy Teams chats from one tenant to another. But you can run a Windows PowerShell script by using CLI for Microsoft 365 plus Graph API. Here’s a step-by-step guide explaining how to pull this off.
Steps for copying chats from one tenant to another
Step 1: We’ll start off by creating a custom Azure ADD app. It can check login permissions and start the login process if the user isn’t logged in yet.
function createCustomAADApp{
param (
[Parameter(Mandatory = $false)]
[string]$APIPermissionList,
[Parameter(Mandatory = $false)]
[string]$AppManifestJSONFile
)
# Checking Login status and initiate login if not logged
$LoginStatus = m365 status --output text
if($LoginStatus -eq "Logged out")
{
Write-Host "Not logged in. Initiating Login process"
m365 login
}
Write-Host "Creating AAD App"
# Create custom App with needed permission
$AddedApp = (m365 aad app add --manifest $AppManifestJSONFile --redirectUris "https://login.microsoftonline.com/common/oauth2/nativeclient" --platform publicClient --apisDelegated $APIPermissionList --output json) | ConvertFrom-Json
return $AddedApp
}
function initiateLoginUsingCustomAddin{
param (
[Parameter(Mandatory = $true)]
[string]$CustomAADAppId,
[Parameter(Mandatory = $false)]
[string]$AppTenantId = ""
)
if($AppTenantId -eq "")
{
$LoginStatus = m365 status --output text
Write-Host $LoginStatus
if($LoginStatus -eq "Logged out")
{
m365 login
}
$AppTenantId = m365 tenant id get
}
# Checking whether current Login ID is using above custom App ID or not. If not, initiating login
$LoggedAppId = ((m365 cli doctor --output json) | ConvertFrom-Json).cliAadAppId
if($CustomAADAppId -ne $LoggedAppId)
{
#login using Custom App ID
Write-Host "Initiating login using Custom Add ID : $CustomAADAppId to Tenant $AppTenantId"
m365 login --appId $CustomAADAppId --tenant $AppTenantId
}
}
Step 2: The next part of our code will help us fetch any Teams conversation we want and export it to CSV.
function getMessagesFromConversation{
param (
[Parameter(Mandatory = $false)]
[string]$ConversationURL,
[Parameter(Mandatory)]
[string]$AccessToken,
[Parameter(Mandatory)]
[string]$ChatID
)
$NextConversationURL = $null
if ([string]::IsNullOrEmpty($ConversationURL))
{
$NextConversationURL = "https://graph.microsoft.com/v1.0/chats/$ChatID/messages?$top=50"
}
else
{
$NextConversationURL = $ConversationURL
}
Write-Host "URL : $NextConversationURL"
$Headers = @{
'Content-Type' = 'application/json'
'Authorization' = 'Bearer ' + $AccessToken
}
$GraphResponse = Invoke-RestMethod -Uri $NextConversationURL -Headers $Headers -Method Get
#Write-Host $GraphResponse.value
$GraphResponse.value | Select-Object @{Name="Sent Date"; Expression={Get-Date $_.createdDateTime -Format "MM/dd/yyyy HH:mm:ss "}}, @{Name="Attendee"; Expression={ $_.from.user.displayName }}, @{Name="Chat Content"; Expression={$_.body.content}}, @{Name="Is Reply"; Expression={$_.attachments.contentType}}, @{Name="Reply Content"; Expression={$_.attachments.content}} | Export-Csv "$PSScriptRoot\ExportedChats.csv" -Append
if($GraphResponse.'@odata.count' -gt 0)
{
getMessagesFromConversation -ConversationURL $GraphResponse.'@odata.nextLink' -AccessToken $AccessToken -ChatID $ChatId
}
}
Step 3: Next, write the following code to get the custom app ID with the Graph API permissions.
$APIPermissionList = "https://graph.microsoft.com/User.Read,https://graph.microsoft.com/Chat.Read,
https://graph.microsoft.com/Chat.ReadWrite"
$AppManifestJSON = "@custom-app-manifest.json"
$AddedApp = createCustomAADApp -APIPermissionList $APIPermissionList -AppManifestJSONFile $AppManifestJSON
Write-Host "AAD App with the details will be used. App ID : $($AddedApp.appId). Object ID : $($AddedApp.objectId)"
$CustomAADAppId = $AddedApp.appId
Step 4: Next, write down the chat ID of the conversation you want to copy. You can find the chat ID by opening the conversation in your browser.
$ChatId = "19:2a1a111e-9b3a-4694-93ca-01e364a79abf_8bc5c293-4208-414e-9837-718a0385be6b@unq.gbl.spaces"
Step 5: Now, you’ll need to pass the tenant ID. If you don’t have a value for this, CLI for M365 will create one for you.
initiateLoginUsingCustomAddin -CustomAADAppId $CustomAADAppId
Step 6: Finally, fetch the access token for the graph API call.
$AccessToken = m365 util accesstoken get --resource https://graph.microsoft.com --new --output text
Write-Host $AccessToken
getMessagesFromConversation -AccessToken $AccessToken -ChatID $ChatId
Step 7: That’s it! Note that this script uses a manifest file to create a custom ADD app. Here’s the file used in the script:
{
"name": "Teams Conversation Export API",
"isFallbackPublicClient": true
}
Use ShareGate to copy chats, teams, and more
And you’re done! Also, you don’t need a global admin role to run this script. However, you do need delegated permissions for Chat.Read and Chat.ReadWrite.
Check out our PowerShell script documentation about copying in Teams using ShareGate, which you can leverage to do more advanced stuff like making incremental copies.
5. How to copy user permissions in SharePoint using Windows PowerShell console
There’s no built-in feature to copy user permissions in SharePoint. This can create issues if you’re trying to clone user permissions at scale, and manual labor is involved in copying user permissions manually.
Luckily, in the PowerShell console, using the ‘Clone-SPUser command’, you can copy user permissions by creating a CSV file and specifying permissions there.
Steps for copying permissions in SharePoint
Let’s look at an example where we copy site permissions to show how this would work.
Step 1: Create a CSV file in the following manner, specifying user permissions as required.
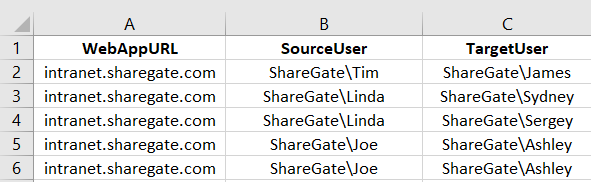
Step 2: In PowerShell ise, specify the CSV file path.
$CSVFilePath = "C:\temp\CloneUsers.csv"
Step 3: Next, import the CSV file.
$CSVFile = Import-Csv $CSVFilePath
Step 4: Finally, read the file and create a document library using the following ForEach loop:
ForEach($Line in $CSVFile)
{
#Get CSV File Entries
$WebAppURL = $Line.WebAppURL
$SourceUser = $Line.SourceUser
$TargetUser = $Line.TargetUser
#Call the Function to Clone user permissions
Clone-SPUser $SourceUser $TargetUser $WebAppURL
}
Easily copy object permissions
Use ShareGate to copy object permissions (site, list, file, or folder) using PowerShell. For more information, check out our PowerShell documentation about copying object permissions.
Skip the PowerShell scripts by using ShareGate to automate it all
With PowerShell commands, you can pull off some neat scripts to automate your Microsoft 365 environment. But PowerShell scripting has certain limitations. And it won’t be the best solution when a task such as copying permissions can be executed by pressing a button.
You can do that with ShareGate, an out-of-the-box solution for managing Microsoft 365, where you achieve maximum efficiency beyond PowerShell scripts.
From automated permissions management of SharePoint and Teams to easy incremental copying, ShareGate has everything built-in.
With a user-friendly UI that helps system administrators cut out repetitive and time-consuming tasks, automating your Microsoft 365 environment becomes a breeze.
Sounds exciting? Cut out future manual labor hours, and take ShareGate out for a test drive to start your automation journey today!
I love writing PowerShell, but if ShareGate has a report or capability that gets me there instead, I can save all that time.
Marc D. Anderson, Microsoft MVP